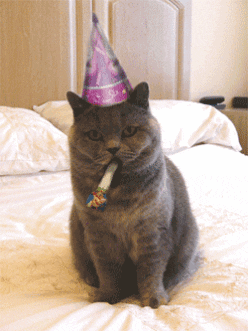
And it’s my Birthday!
Week 4 is here, and this week we are going to learn about Bluetooth and serial data communication. I loved hearing the progress you have been making.
The presentation that I cover can be downloaded here as well.
Step 1: Introduction & What I’ve Built
Update on my desk light project, it now has a temperature reading!
Step 2: Arduino vs. Pictoblox
Our project is going to get a little complicated so we need to use the Arduino IDE and write some real code. But don’t worry, we’ll do this together.
Step 3: Installing the Arduino IDE
If you are going to use the installed version of Arduino, this video will cover the setup. If you are going to use the online version, you could skip this video.
Step 4: Using the On-line Arduino IDE
If you are going to use the on-line version of the Arduino IDE, this video will walk you through the process. If you downloaded the Arduino IDE in step 3, you can skip this video.
Step 5: Arduino IDE Overview
Let’s take a few minutes and review the Arduino IDE so you know where to find everything.
Step 6: Another Blinkie!
In this lesson, we are going to revisit the Blinkie program with the Arduino IDE instead of PictoBlox.
Step 7: Writing Blinkie Using Arduino IDE
Using the Arduino IDE, let’s “write” some Arduino code…but only with a few clicks of the mouse.
Step 8: Let’s Write Blinkie from Scratch
And, let’s write some real code! We will write code using the C programming language.
Step 9: HC-06 Bluetooth Module
Let’s move on to our Bluetooth module, this will be very interesting.
Step 10: Building the Bluetooth Circuit
Time to wire up this our Bluetooth module on the breadboard and learn a bit about the wiring.
Step 11: Time For Some Bluetooth Code
Writing a little code, but you can just copy paste it if you want. (It’s below the video)
#include <SoftwareSerial.h>
SoftwareSerial BT(A0, A1);
void setup() {
Serial.begin(9600);
BT.begin(9600);
pinMode(13, OUTPUT);
pinMode(12, OUTPUT);
pinMode(11, OUTPUT);
pinMode(10, OUTPUT);
}
void loop() {
if (BT.available())
{
char command = BT.read();
Serial.println(command);
if (command == '1')
{
digitalWrite(10, HIGH);
} else if (command == '2') {
digitalWrite(11, HIGH);
} else if (command == '3') {
digitalWrite(12, HIGH);
} else if (command == '4') {
digitalWrite(13, HIGH);
} else if (command == '0') {
digitalWrite(10, LOW);
digitalWrite(11, LOW);
digitalWrite(12, LOW);
digitalWrite(13, LOW);
}
}
}
Step 12: TX & RX Explained
I wanted to explain how the serial communication method works. But, if you don’t really care, you can skip this video.
Step 13: And That’s a Wrap
Whew, we made it. It was a long journey but we made it. Next week, let’s build our car’s chassis.